How to use Microsoft Graph API with Laravel
By Dillon Smart · · · 0 Comments
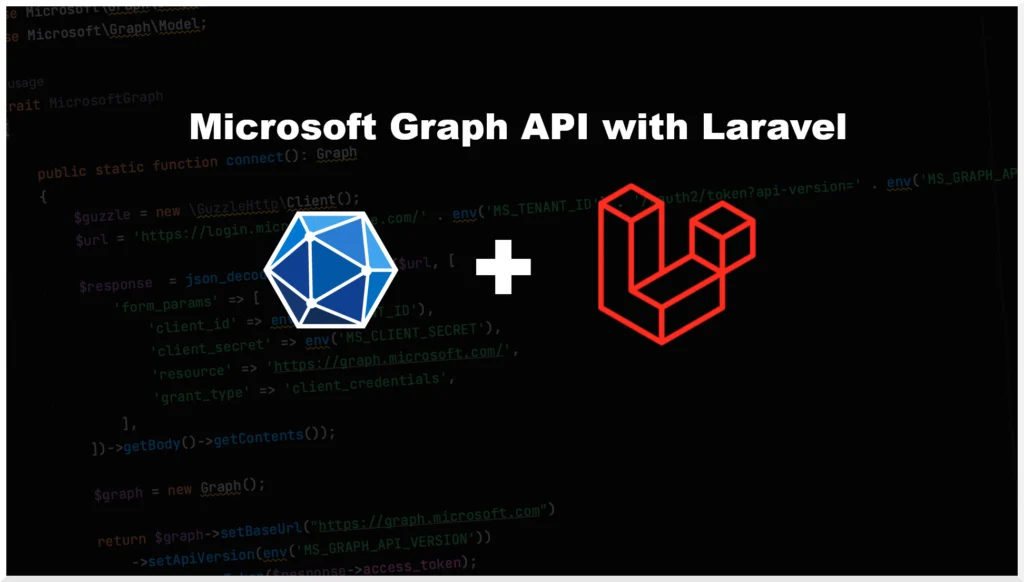
Microsoft Graph is a gateway to data and intelligence within Microsoft 365. Microsoft Graph is great to build apps for organizations and consumers using the vast amount of data stored in Microsoft 365. In this post, we are going to integrate with Microsoft Graph API in Laravel to get all users assigned to your tenant.
You will need to have already created an Azure App Registration to follow along with this post. If you are unsure how to create an Azure App Registration, take a look at this Quickstart Guide by Microsoft which will take you through the process.
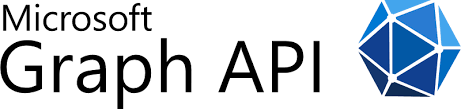
Microsoft has created an open-source SDK for PHP on GitHub. Install the SDK using Composer.
composer require microsoft/microsoft-graph
Or you can edit your composer.json file:
{
"require": {
"microsoft/microsoft-graph": "^1.57.0"
}
}
To make requests to Microsoft Graph API we will be using GuzzleHTTP client. It’s a great package that makes it easy to send HTTP requests and integrate with web services. Also, Guzzle comes installed with Laravel, which is great.
I have created a Trait to help with this example. To install the Trait, under the App directory, create a new empty directory called “Integrations“. Download the Trait from Gist, and place the file within the App/Integrations directory.
Setup Microsoft Graph API in Laravel
To get started setting up Microsoft Graph API with Laravel, keys to the .env file in the root of your Laravel application.
MS_TENANT_ID=
MS_CLIENT_ID=
MS_CLIENT_SECRET=
MS_GRAPH_API_VERSION=v1.0
How to find your Microsoft Tenant ID
To find your Microsoft Tenant ID, log in to Microsoft Azure. From the menu, select Azure Active Directory > Overview. You can find the Tenant ID within the Overview tab.
How to find your Microsoft Azure App Client ID and Client Secret
To find your Client ID, from the same menu in Azura Active Directory, select App Registrations > Your App > and Client Secrets, which can be found under Essentials. The Client Secret for your Azure App is only available at the creation of the app and cannot be retrieved afterward.
Setup Azure app permissions
We will be getting a list of users in the tenant. The permissions required to make this API request are Application Permissions.
- User.Read.All
List all users using Microsoft Graph API
To help with Microsoft Graph in Laravel, I created a simple Trait to connect and list users within your Microsoft 365 tenant. This can be found on GitHub.
Use App\Integrations\MicrosoftGraph;
Return MicrosoftGraph::users();
You can get familiar with Microsoft Graph API by using Graph Explorer created by Microsoft to test different endpoints and responses.
Was this post helpful? Please leave a comment below.
0 Comment