How to check Composer version – Install and use Composer for PHP
By Dillon Smart · · · 1 Comments
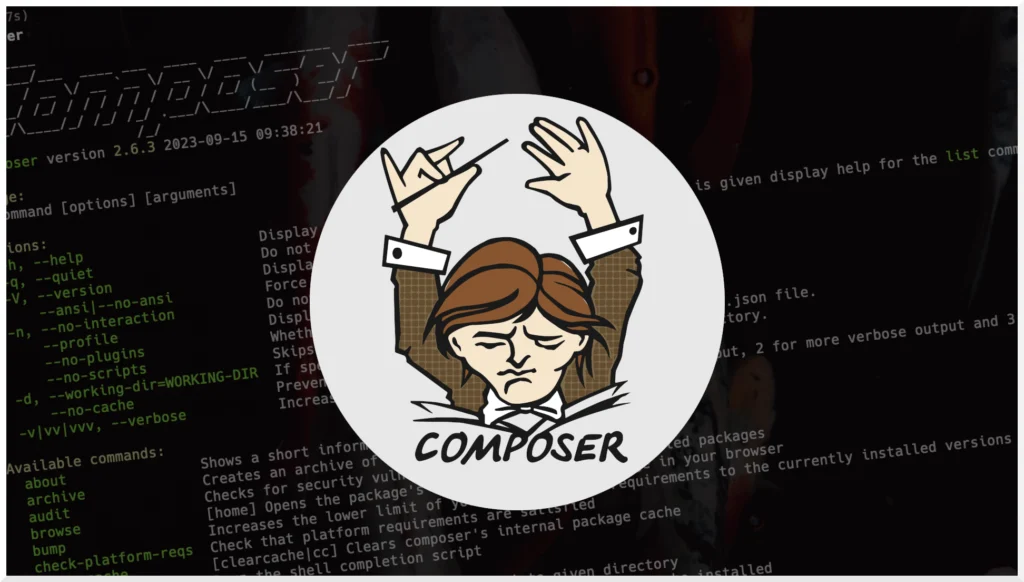
Composer is a dependency manager for PHP. First released in 2012, Composer, and its adoption by popular frameworks such as Laravel has single-handedly driven the rise in PHP adoption in recent years. In this post, I will show you how to install and use Composer.
How to install Composer
To get started, download Composer from the official website’s download page.
To install Composer, follow the sets outlined in the documentation.
Copy the commands from the website and execute them in a terminal. To begin with, Composer will be installed in the current directory. Later, we will move the composer.phar file to a directory in your PATH to be used system-wide.
Composer install commands
php -r "copy('https://getcomposer.org/installer', 'composer-setup.php');"
php -r "if (hash_file('sha384', 'composer-setup.php') === '906a84df04cea2aa72f40b5f787e49f22d4c2f19492ac310e8cba5b96ac8b64115ac402c8cd292b8a03482574915d1a8') { echo 'Installer verified'; } else { echo 'Installer corrupt'; unlink('composer-setup.php'); } echo PHP_EOL;"
php composer-setup.php
php -r "unlink('composer-setup.php');"
The hash will change, so ensure you use the commands from the official website.
How to install Composer globally
To install Composer globally, you need to move the composer.phar file to a directory in your PATH so you can call Composer globally from the terminal.
sudo mv composer.phar /usr/local/bin/composer
To test the install worked correctly, try running the composer command from the terminal.
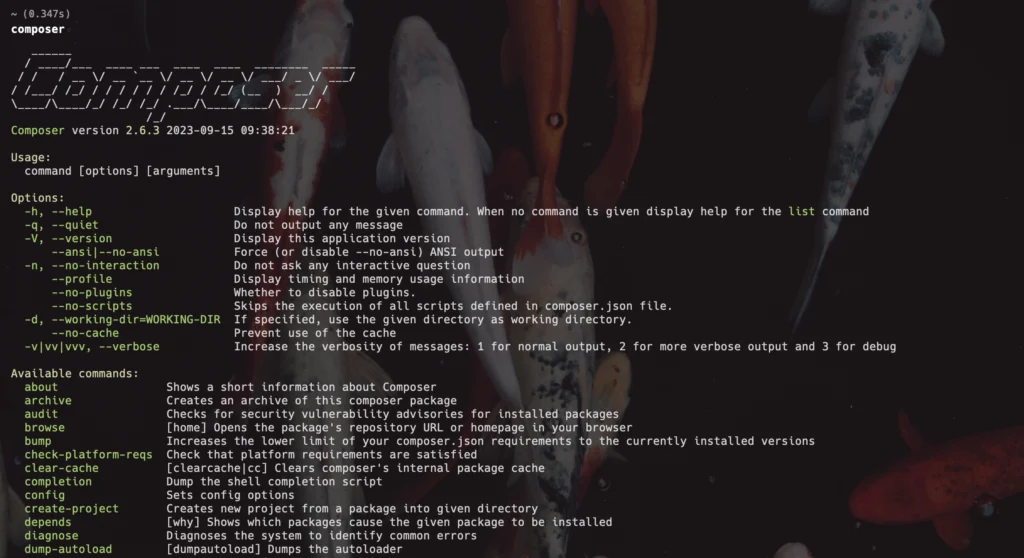
How to check which Composer version is installed
To check which composer version is installed on your machine, from the current path run the composer -V command.
composer -V
This works on all devices, so you can check the installed Composer version on macOS, Windows, and Linux by running the composer -V
command.
How to update Composer to version 2
In 2020, Composer 2.0 was released. Version 2.0 came with several improvements over version 1 such as:
- Speed improvements
- Error reporting improvements
- Platform checks during runtime
To update Composer to version 2, run the following command in the terminal.
composer self-update --2
If you don’t have Composer in your PATH, you will need to run:
composer.phar self-update --2
How to downgrade Composer to version 1
If you encounter issues with version 2.0 or are using an older version of a Composer framework, you may need to downgrade to version 1. To downgrade to version 1 run the following in the terminal.
composer self-update --1
If you don’t have Composer in your PATH, you will need to run:
composer.phar self-update --1
How to use Composer
To use Composer in your projects all you need is a composer.json file in the root directory of your project. This file describes the dependencies required for your project.
First, head over to Packagist to find the packages you want to use in your project.
In the composer.json file, specify the “require” key. Within the “require” object, add the package name and its version constraint.
In this example, we will be checking our platform for PHP 8.0 and installing the Microsoft Graph PHP SDK.
{
"require": {
"php": "8.0",
"microsoft/microsoft-graph": "^1.35",
}
}
Difference between Composer Install and Composer Update
Composer Update
To install your dependencies, first, you should run composer update
. This creates a composer.lock file, which locks your project to the specified package versions. After creating the lock file composer will run checks and install the dependencies in the lock file to the vendors/ directory.
You should commit the lock file to your repo so any other developers who are working on the same project are also locked to the specified dependency versions.
Composer Install
To install other dependencies, you can either add them manually to your composer.json file or install them directly from the terminal:
composer install microsoft/microsoft-graph
The above command will install the latest version of the Microsoft Graph PHP SDK.
When working on a project that already has a composer.lock file, you should run composer install.
Install a specific version of a package with Composer
If you need to install a specific version of a package with composer, you can add the version number of the package to the composer require
command:
composer require microsoft/microsoft-graph:1.74.0
Your lock file does not contain a compatible set of packages
It is common to encounter a platform requirements error with Composer.
Your lock file does not contain a compatible set of packages please run composer update.
A way to avoid this error is to use the ignore platform requirements option on the command.
composer install --ignore-platform-reqs
Or
composer update --ignore-platform-reqs
Conclusion
Composer has helped bring PHP up to standard with other programming languages by providing a convenient way of installing and managing packages. To learn more about Composer, check out this Composer Cheatsheet.
Next, learn how to change your PHP version.
1 Comment
[UPDATED 2022] Change PHP version on Ubuntu, Linux - IKnowThatNow
[…] Next, learn how to install Composer and check your Composer version. […]