JavaScript Spread & Rest Operators
By Dillon Smart · · · 0 Comments
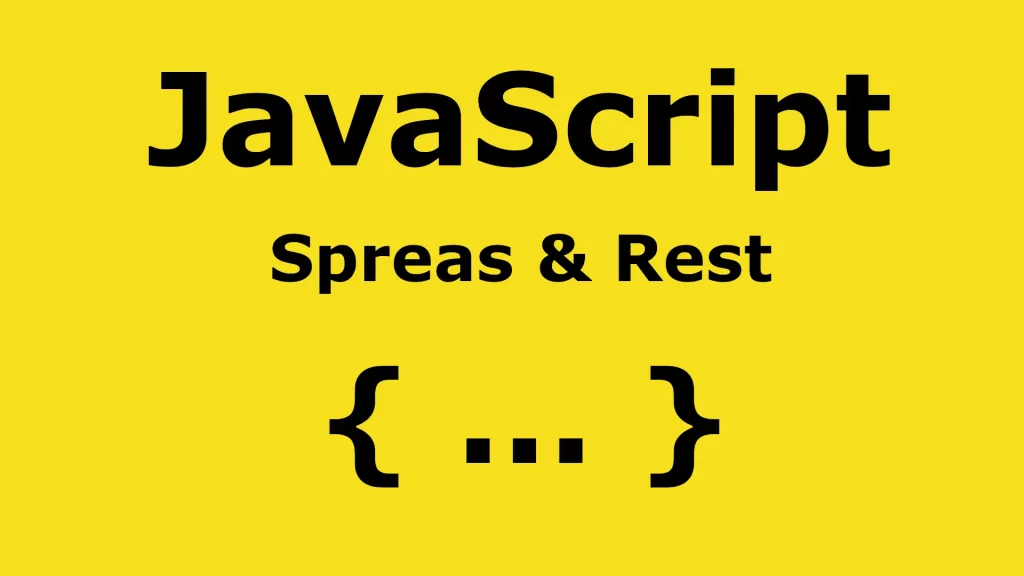
In JavaScript, three dots ( … ) are used for both the Spread and Rest operators. Spread and Rest perform different actions. Let’s learn what the JavaScript Spread & Rest Operators do.
JavaScript Spread Operator
The JavaScript Spread Operator ( … ) allows us to copy and split an Array or Object into another Array or Object.
Let’s look at an example of the Spread Operator in use:
const numbers = [1, 2, 3]; const newNumbers = [4, 5, 6]; const joinNumbers [...numbers, ...newNumbers]; console.log(joinNumbers); // Output: [1, 2, 3, 4, 5, 6]
JavaScript Rest Operator
In JavaScript, the Rest Operator is used in a very different way to the Spread Operator. The Rest Operator puts the rest of some specific values into an array.
What does this mean?
The Rest Operator allows a function to accept an indefinite number of arguments as an array.
Let’s look at an example of the Rest Operator in use:
function sumOfNumbers(...numbers) { let value = 0; for(const num of numbers) { total += num; } return total; } console.log( sumOfNumbers(1, 2, 3) ); // Output: 6 console.log( sumOfNumbers(5, 10, 15, 20) ); // Output: 50
Conclusion
The JavaScript Spread & Rest Operators can prove to be very useful for your web applications.
Learn about Destructuring in JavaScript.
0 Comment