Destructuring in JavaScript
By Dillon Smart · · · 0 Comments
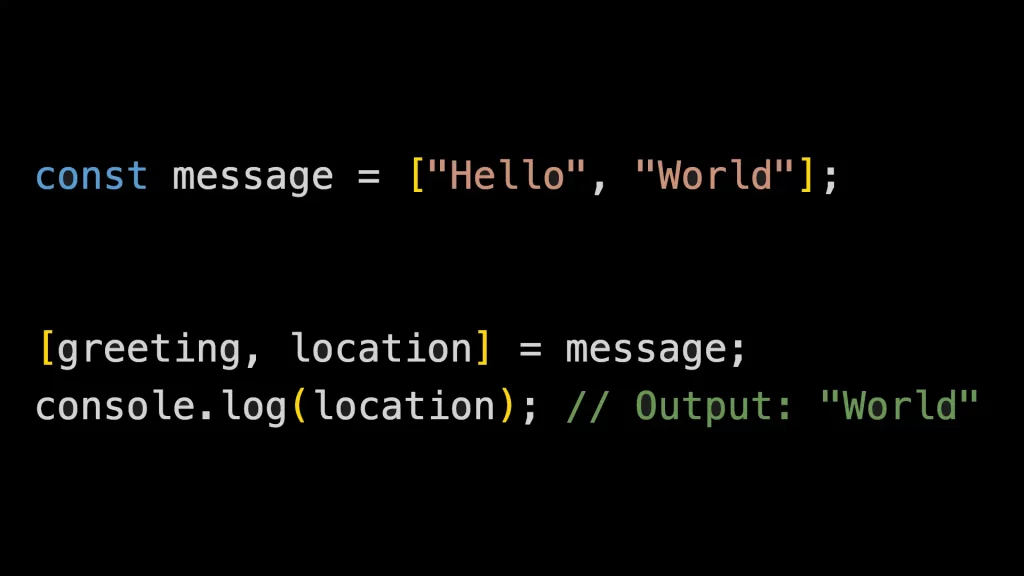
Introduced in ES6, Destructuring in JavaScript is a way to unpack values from arrays, or properties from objects, into distinct variables.
What is a use-case of Destructuring in JavaScript?
Until ES6, if we wanted to extract data from an array we would have to do something similar to:
let message = ["Hello", "Dillon"]; let greeting = message[0]; let name = message[1]; console.log(greeting); // Output: "Hello" console.log(name); // Output: "Dillon"
How to use Destructuring in JavaScript
If we want to extract data from an array, using Destructuring in JavaScript we can do it a lot easier.
Using the same example as above, but this time we will use the Destructuring Assignment.
let message = ["Hello", "Dillon"]; let [greeting, name] = message; console.log(greeting); // Output: "Hello" console.log(name); // Output: "Dillon"
Declaring variables before assignment
With the destructuring assignment, we can also declare the variables before they are assigned.
let greeting, name; let [greeting, name] = ["Hello", "Dillon"]; console.log(greeting); // Output: "Hello" console.log(name); // Output: "Dillon"
We can also skip items in the array like so:
let [greeting,,msg] = ["Hello", "Dillon", "Welcome"]; console.log(greeting); // Output: "Hello" console.log(msg); // Output: "Welcome"
Conclusion
Many features introduced in ES6 can be overlooked.
Learn about Spread & Rest Operators in JavaScript.
0 Comment