Alternative ways of doing the same things in Laravel
By Dillon Smart · · · 1 Comments
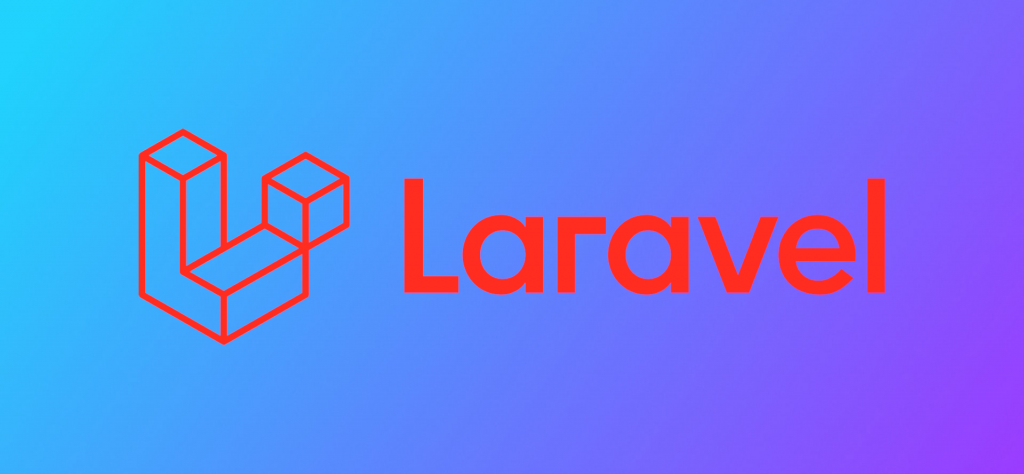
Laravel is a large framework, with contributions coming from almost 2000 developers worldwide. Over its 10 year journey, there have been additions to the framework to do the same thing, only shorter. In this post, I have highlighted some alternative ways of doing the same things in Laravel.
- How to get the authenticated users id
- How to clear cache in Laravel
- Return a route view
- PHP isset function
Get the authenticated users id in Laravel
There are a number of different ways of retrieving the authenticated user’s id in Laravel. Below are some of the most common ones.
auth()->user()->id
or
\Auth::user()->id
or you can use the shorter way
auth()->id()
How to clear cache in Laravel
Laravel provides a few commands to clear cache files from the filesystem. The commands below each target a different cache:
php artisan cache:clear php artisan config:clear php artisan route:clear
But did you know you can clear all caches with one command!
php artisan optimize:clear
Route views in Laravel
I have seen this a number of times in projects I have either inherited or projects I have helped junior developers with. Routes that call a controller method, but only call a static view. There is a simpler way of doing this, and it’s been there since Laravel 5.5 which was released in 2017!
Route::view('/', 'front.pages.home.index')->name('website-home');
Do you know of an alternative way of doing something in Laravel? Share it below!
PHP isset function
This isn’t strictly related to Laravel. In PHP isset function determines if a variable is declared and different than null. The isset function supports multiple variables.
if(isset($var1) && isset($var2)) { // variables are declared }
A cleaner solution would be:
if(isset($var1, $var2)) { // variables are declared }
1 Comment
Laravel 9: How to Add a New Column to an Existing Table in a Migration - IKnowThatNow
[…] has a number of features designed to make developing web applications easier and faster. Migrations in Laravel allows us to […]