SOLID Principles of Object-Oriented Design
By Dillon Smart · · · 1 Comments
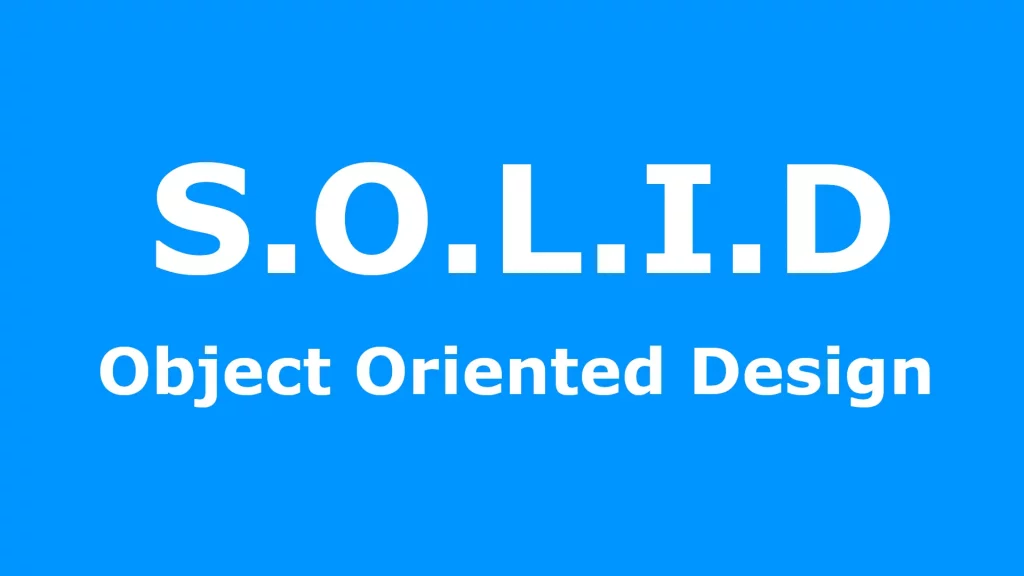
When building software it’s important to follow best practices and adhere to principles set out by industry leaders. In this post, we will explore the SOLID Principles.
SOLID stands for:
- S – Single-responsibility principle
- O – Open-closed Principle
- L – Liskov Substitution Principle
- I – Interface Segregation Principle
- D – Dependency Inversion Principle
Great! But what does all that mean?
What does SOLID mean?
Let’s look at each of the principles of the SOLID acronym.
Single-Responsibility Principle of Object-Oriented Design
A class should have one and only one reason to change, meaning that a class should have only one job.
Single Responsibility is the most important of the SOLID principles. The principle refers to not only single responsibility in your code, but also in your files.
Here are some criteria to judge if your code compiles with the Single Responsibility Principle:
A single file should contain a single class
This should be commonplace as many frameworks already advise this in their style guides. Classes and file names should be related. I.e. UserController.php should contain the class UserController.
The class should only perform one task
The Class and File name should be related and therefore should perform actions that relate. I.e. UserController class should only contain functions that are related to Users.
Functions (or methods) should only do one thing
The logic of a function should relate to the name of the function. Here is an example of bad practice.
public function getUsersAndUpdateVerified() { $users = User::where('age', '>=', 18) ->orderBy('name', 'ASC') ->get(); foreach($users as $user){ $user->verified = true; $user->save(); } }
As you can see, in the function above there are two actions taking place. This code should be updated to:
public function getUsersOverEighteen() { return User::where('age', '>=', 18) ->orderBy('name', 'ASC') ->get(); } public function verifyUsers() { $users = $this->getUsersOverEighteen(); foreach($users as $user){ $user->verified = true; $user->save(); } }
Small comment blocks
If you are finding yourself needing to write large comment blocks explaining the logic of a function, then the function is too ambiguous and therefore violates the principle.
The Open-Closed Principle
The Open-Closed Principle in OOP states that classes, modules, or functions should be open for extension, but closed to modifications.
In the real world when building software, requirements that are set initially will most likely change in the future. You should build your software in such a way that the entity can be extended, but not modified as these requirements change.
The Open-Closed principle helps prevent legacy code from breaking.
Liskov Substitution Principle
The Liskov Substitution Principle (LSP) states that objects of a superclass shall be replaceable with objects of its subclasses without breaking the application.
Objects of your subclasses, classes that extend your superclass, should behave in the same way as the objects in your superclass.
To achieve LSP in your codebase is to ensure methods in your subclasses accept the same input parameter values as the method of the superclass.
LSP can be hard to implement and enforce, especially in legacy codebases that haven’t followed the principle. The best way to enforce LSP is through code reviews.
Interface Segregation Principle
Similar to the Single Responsibility Principle, the Interface Segregation Principle is used to reduce the effects of requirement changes by splitting the software into smaller, independent parts, like microservices.
Dependency Inversion Principle
In OOP, the Dependency Inversion Principle is a methodology for loosely coupling software modules (classes, functions).
The principle favors abstraction.
Conclusion
It’s important to follow best practices and principles when building software. Not only does it make it easier to scale the product, but it makes life easier for other software developers and yourself in the future.
Learn about DRY.
1 Comment
DRY: What is DRY in Development - IKnowThatNow
[…] Learn about the SOLID Principle. […]